![]() |
An application needs to consume Messages as soon as they’re delivered.
How can an application automatically consume messages as they become available?
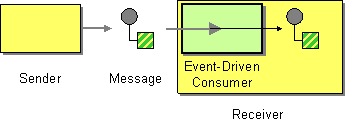
The application should use an Event-Driven Consumer, one that is automatically handed messages as they’re delivered on the channel.
This is also known as an asynchronous receiver, because the receiver does not have a running thread until a callback thread delivers a message. We call it an Event-Driven Consumer because the receiver acts like the message delivery is an event that triggers the receiver into action.
An Event-Driven Consumer is an object that is invoked by the messaging system when a message arrives on the consumer’s channel. The consumer passes the message to the application through a callback in the application’s API. (How the messaging system gets the message is implementation-specific and may or may not be event-driven. All the consumer knows is that it can sit dormant with no active threads until it gets invoked by the messaging system passing it a message.)
... Read the entire pattern in the book Enterprise Integration Patterns
Example: RabbitMQ ConsumerNEW
RabbitMQ consumers are by default event-driven. When consuming messages off a Channel, you have to provide an implementation of the Consumer interface. The following code example sets up a very simple consumer that simply prints incoming messages as strings:
Consumer consumer = new DefaultConsumer(channel) { @Override public void handleDelivery( String consumerTag, Envelope envelope, AMQP.BasicProperties properties, byte[] body) throws IOException { String message = new String(body, "UTF-8"); System.out.println("Received '" + message + "'"); } }; boolean autoAck = true; channel.basicConsume(queueName, autoAck, consumer);
This implementation is not a Transactional Client because the client acknowledges messages automatically on receipt but before processing.
The complete source code for this example can be found on Github.
Related patterns:
Competing Consumers, Durable Subscriber, Message, Message Channel, Message Dispatcher, Selective Consumer, Polling Consumer, Transactional Client